After reading this blog, you should be able to grasp what an Amazon API Gateway is, its architecture, benefits of it, how it operates and how to get start Amazon API Gateway with http API
What is Amazon API Gateway?
Amazon API Gateway is a web service that allows developers to easily construct, publish, maintain, monitor, and protect APIs at any scale.
This gateway is in charge of accepting and processing hundreds of thousands of concurrent API calls.
Amazon API Gateway makes it simple to administer APIs that allow applications to connect to your backend services. Use this tool to create RESTful APIs or WebSocket APIs with real-time updates that allow apps to communicate with one another.
AWS recently announced AWS HTTP APIs. These are an alternative to the API Gateway REST APIs, which we cover in our blog to AWS HTTP APIs. Many Serverless applications use Amazon API Gateway, which conveniently replaces the API servers with a managed serverless solution.
Architecture of API Gateway
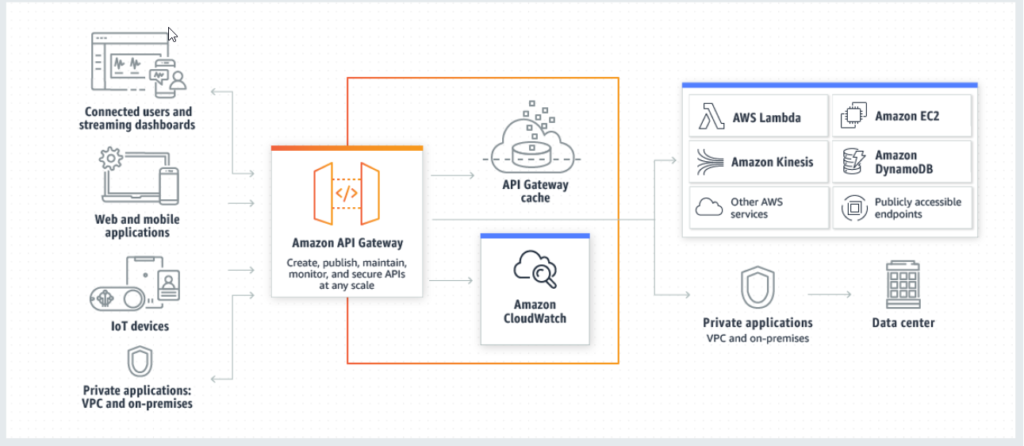
This diagram shows how the APIs you create in Amazon API Gateway provide an integrated and uniform developer experience for building AWS serverless applications for you or your developer customers. API Gateway manages all aspects of accepting and processing hundreds of thousands of concurrent API calls. Traffic management, permission and access control, monitoring, and API version management are examples of these duties.
API Gateway serves as a “front door” for applications to access data, business logic, or functionality from your backend services, such as Amazon Elastic Compute Cloud (Amazon EC2) workloads, AWS Lambda code, any web site, or real-time communication applications.
Features of Amazon API Gateway
Amazon API Gateway includes numerous features and functions, including:
Security: To protect your API from harmful assaults, use security technologies like as SSL/TLS encryption, OAuth 2.0, and native OIDC. Using AWS Identity Access Management (IAM) and Amazon Cognito, you can ensure that only authorized and authenticated users have access to your API.
Traffic management: Use features such as rate restriction to keep your API from becoming overloaded with requests.
Amazon CloudWatch: View performance metrics and API call statistics from a dashboard, providing you with complete visibility into your services.
Versioning: Manage multiple API versions independently to develop new features and upgrade old ones more effectively.
How to Access API Gateway?
Amazon API Gateway can be accessed in the following ways:
AWS Management Console – The AWS Management Console is a web interface that allows you to create and manage APIs. You can access the API Gateway via amazon console. after completing the steps in Prerequisites for Getting Started with API Gateway.
AWS SDKs – If you’re using a programming language for which AWS has an SDK, you can use it to access API Gateway. SDKs make authentication easier, connect with your development environment, and provide you access to API Gateway operations. See Tools for Amazon Web Services for further details.
AWS Command Line Interface and AWS Tools for Windows PowerShell are other accessing ways
How API Gateway is useful for the Serverless ecosystem?
API Gateway is the component that connects Serverless functions and API specifications in the Serverless ecosystem. The ability to directly initiate the execution of a Serverless function in response to an HTTP request is the primary reason API Gateway is so valuable in Serverless setups: it enables a completely serverless architecture for web applications. When combined with other AWS services, API Gateway allows you to create a completely functional customer-facing web application without having to manage a single server.
This extends the serverless model’s benefits of scalability, easy maintenance, and low cost due to reduced overhead to popular online applications.
Getting started with API Gateway
You develop a serverless API in this getting started exercise. Instead of spending time deploying and managing servers, serverless APIs allow you to focus on your applications. To begin, use the AWS Lambda console to construct a Lambda function. The API Gateway console is then used to construct an HTTP API. Then you call your API.
When you call your HTTP API, API Gateway forwards the call to your Lambda function. Lambda executes the Lambda function and responds to API Gateway. You will then receive a response from API Gateway.
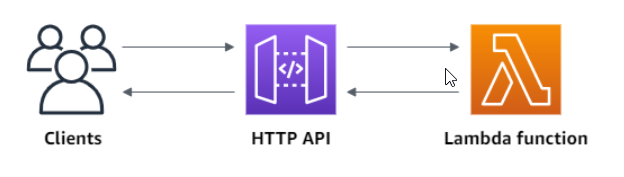
To conduct this exercise, you will need an AWS account and an AWS Identity and Access Management user with console access, as well as the instructions outlined below.
Step 1: Create a Lambda function
You can use a Lambda function as the API’s backend. Lambda executes your code only when it is required and automatically scales from a few requests per day to thousands per second.
In this example, you use the Lambda console’s default Node.js function.
To create a Lambda function:
- Sign in to the Amazon Lambda console.
2. Select the Create function.
3. Enter my-function as the function name.
4. Select the Create function.
The example function responds to clients with a 200 and the words Hello from Lambda!. You can change your Lambda function as long as the function’s answer conforms to the API Gateway standard.
The default Lambda function code should look something like this:
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify('Hello from Lambda!'),
};
return response;
};
We will be able to create the lambda function after this step.
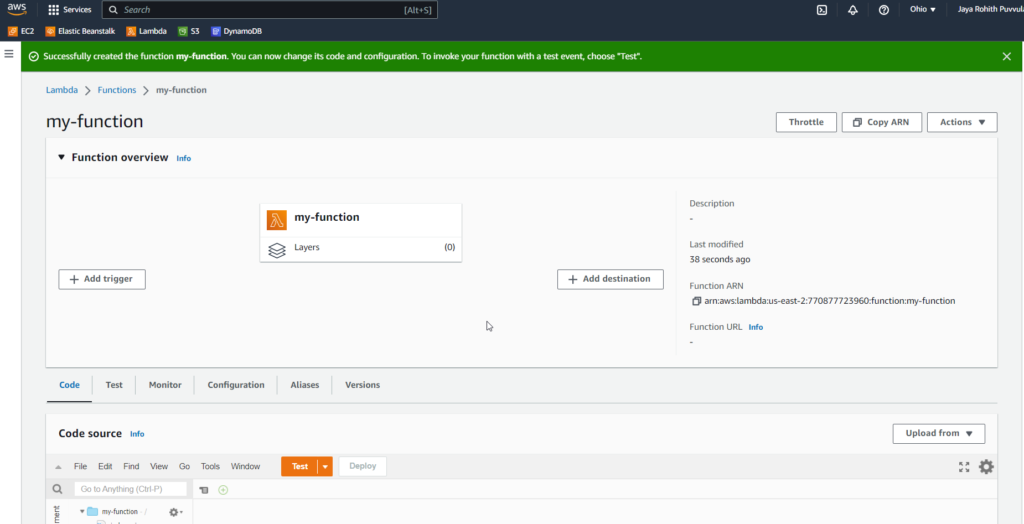
Step 2: Create an HTTP API
You then construct an HTTP API. API Gateway also supports REST APIs and WebSocket APIs, however for this exercise, an HTTP API is the best option. REST APIs support more functionalities than HTTP APIs, although such characteristics are not required for this activity. HTTP APIs are created with little functionalities so that they can be sold at a reasonable cost. WebSocket APIs retain persistent connections with clients in order to support full-duplex communication, which is not necessary in this scenario.
Your Lambda function will have an HTTP endpoint thanks to the HTTP API. Clients are routed to your Lambda function by API Gateway, which subsequently returns the function’s answer.
To create an HTTP API:
- Sign in to the API Gateway console.
- Do one of the following:
- Choose Build for HTTP API to construct your first API.
- If you’ve previously developed an API, select Create API and then Build for HTTP API
- .Select Add integration from the Integrations menu.
- Select Lambda.
- Enter my-function for Lambda function.
- Enter my-http-api as the API name.
- Select Next.
- Review and Create.
You have now developed an HTTP API with a Lambda integration that is ready to handle client queries.
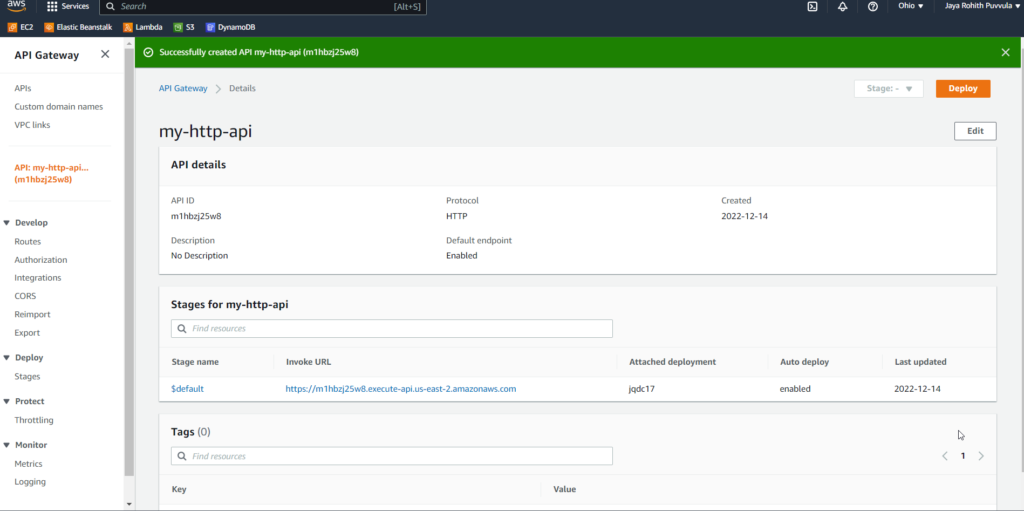
Step 3: Test your API
After that, you test your API to ensure that it is operational. To make things easier, utilize a web browser to access your API.
To test your API
- Sign in to the API Gateway console.
- Choose your API.
- Copy your API’s invoke URL, and enter it in a web browser. Append the name of your Lambda function to your invoke URL to call your Lambda function. By default, the API Gateway console creates a route with the same name as your Lambda function,
my-function
. The full URL should look likehttps://abcdef123.execute-api.us-east-2.amazonaws.com/my-function
.Your browser sends aGET
request to the API. - Verify your API’s response. You should see the text
"Hello from Lambda!"
in your browser.
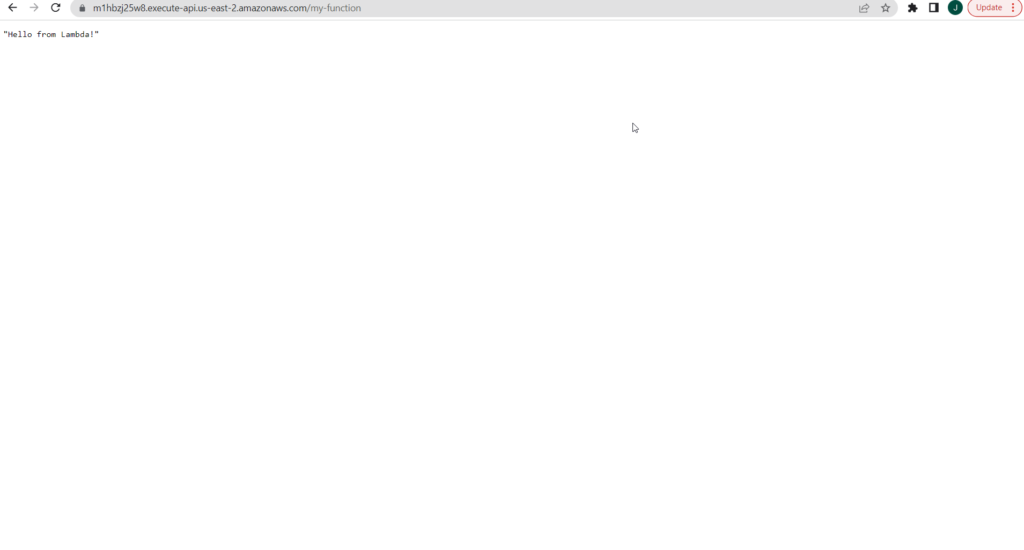