EC2 is the main computational element of the technological stack among the wide range of services that Amazon provides. By offering safe and scalable compute resources in the cloud, EC2 actually makes life simpler for developers. It substantially simplifies the process of scaling up or down, can be linked with a number of other services, and has a pricing structure where you only pay for the usage you receive.
What is AWS Lambda?
AWS Lambda is a serverless computing service provided by Amazon Web Services (AWS). Users of AWS Lambda write functions—self-contained programs written in one of the supported languages and runtimes—and upload them to the service. AWS Lambda then executes the functions in a swift and adaptable way.
The Lambda functions can handle any type of computation, including providing web pages, processing data streams, calling APIs, and integrating with other AWS services. You can trigger Lambda from over 200 AWS services and software-as-a-service (SaaS) applications and only pay for what you use.
In this blog we will look at creating an EC2 instance using AWS Lambda function.There are other ways of creating EC2 instance such as using AWS management console or AWS Command Line Interface. Detailed instructions to create an EC2 instance using console can be found here. Link
Creating Key Pair
When connecting to an Amazon EC2 instance, you must utilize a key pair, which consists of a public key and a private key, to establish your identity. The private key is kept by you, while the public key is kept by Amazon EC2 on your instance. You can safely SSH access your Linux instance using the private key. So before we create the EC2 Instance we will have to create an key pair which will be used when logging into the EC2 Instance. It’s crucial that you keep your private key in a secure location because anyone in possession of it can connect to your instances.
You can create the EC2 key pair using AWS console or use any third-party app to create the key pair and import the key pair in AWS console. For our blog we will create the key pair in the AWS console with key name lambda-ec2-creation.
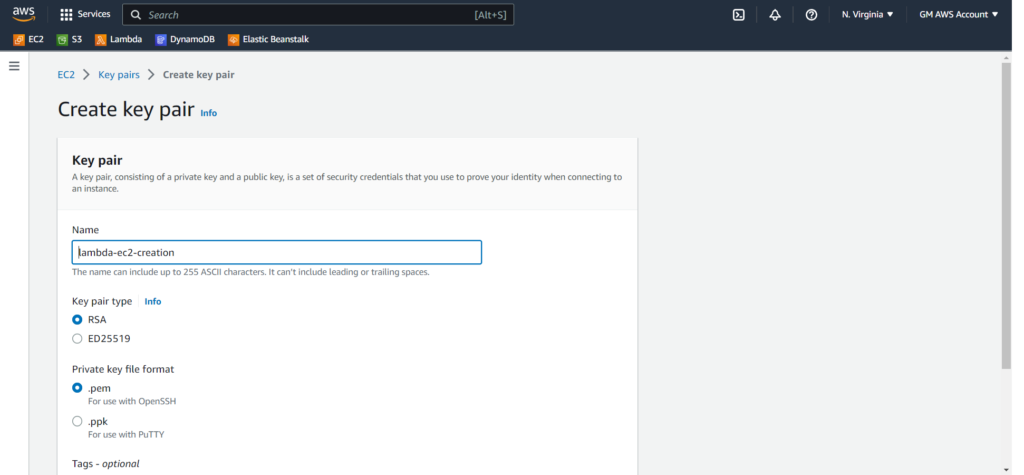
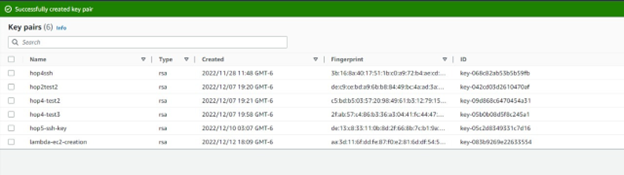
Step 1:
Once you have created the new key pair, go to AWS lambda service on the management console and create a new function. You can choose the runtime environment, I am choosing Python 3.7 . For the Execution role select Create a new role with basic Lambda Permissions options and click on Create Function option.
You can also choose to use an existing role if you already have a role that has permissions to write logs in the Cloudwatch and permissions to create an EC2 instance.
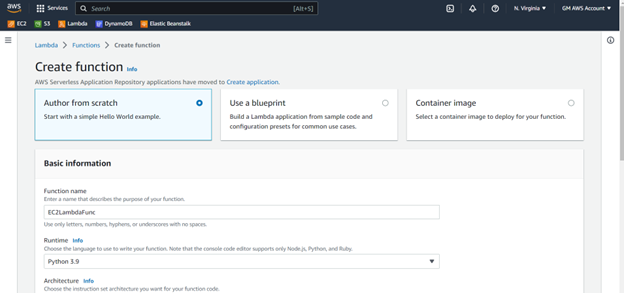
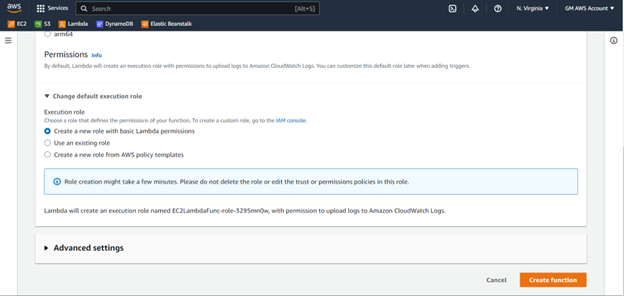
We have created a new function named EC2LambdaFunc.
Step 2:
We will now have to give the IAM role created with EC2LamdaFunc permissions to create an Instance. For doing this navigate to the IAM service in the console and select the Roles option. You will be able to view the role starting with EC2LambdaFunc.When you click on it you will be taken to the permissions screen. Click on Edit policy and replace the json with the following json.
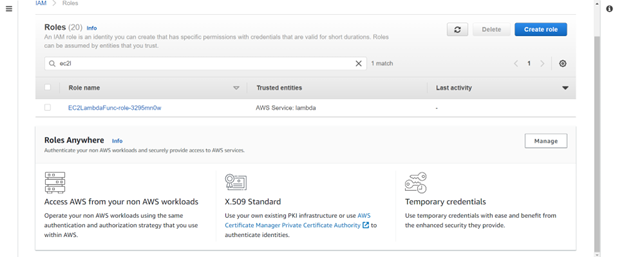
{
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Action": [
"logs:CreateLogGroup",
"logs:CreateLogStream",
"logs:PutLogEvents"
],
"Resource": "arn:aws:logs:*:*:*"
},
{
"Action": [
"ec2:RunInstances"
],
"Effect": "Allow",
"Resource": "*"
}
]
}
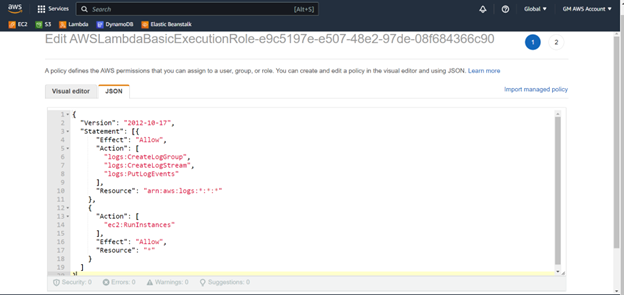
Review the changes and save the policy
Step 3:
Go to the Lambda services in your console and select your function. In the code table paste the following code.
import os
import boto3
AMI = os.environ['AMI']
INSTANCE_TYPE = os.environ['INSTANCE_TYPE']
KEY_NAME = os.environ['KEY_NAME']
SUBNET_ID = os.environ['SUBNET_ID']
ec2 = boto3.resource('ec2')
def lambda_handler(event, context):
instance = ec2.create_instances(
ImageId=AMI,
InstanceType=INSTANCE_TYPE,
KeyName=KEY_NAME,
SubnetId=SUBNET_ID,
MaxCount=1,
MinCount=1
)
print("New instance created:", instance[0].id)
Using Import.os we are fetching the environment variables that we are going to add in the next step.
Using Import boto3 we will be able to access all the services that this SDK provides.In this program we are going to utilize the create-instances method from the ec2 resource boto3.
We are calling the function ec2.create-instances by passing all the config variable values and saving the results in a variable called instance.
The max and min count are used to define the number of instances that you want to create with this program.
For every AWS account there is max limit of the number of instances that can launched. If the max-count variable value is more than this limit then the program will launch the maximum number above the min count.
For our blog we are just creating 1 instance. So we keep both the values 1 for these variables.
We are also printing the launched Instance ID value in the logs using the print statement in the last line.
Save the code by clicking on deploy.
Step 6: We will now define the configuration of the EC2 instance that we are going to create such as the AMI value, Instance-type, Key-Name, Subnet-Id in the environment variables such that these values are not hard coded in the program and the function can be reusable by changing these variable values.
AMI Image value can be found by selecting the Machine of your choice in Launch instance option. For this blog I am using a Ubuntu 20.04.
We are using t2.micro for this blog as it comes with free tier. You can select the instance type of your choice and add it in this variable value.
For Key-Name variable you can paste the name of the key pair that we created in step 1 or any existing key pair in your AWS console.
For Subnet-Id, you can pick any public subnet available in the VPC subnet service dashboard.
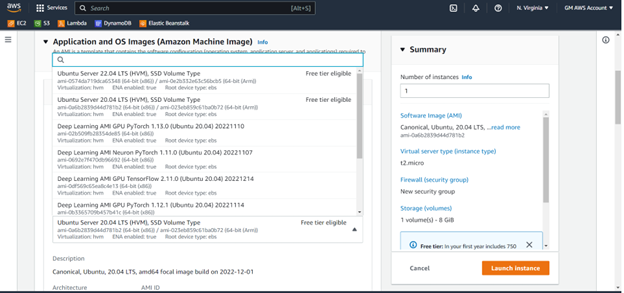
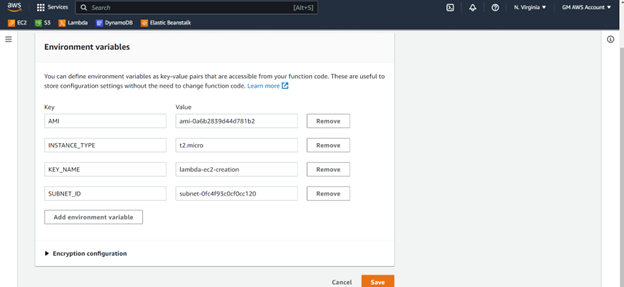
Under the configuration tab select Environment variables and add the above variables.
Step 4:
Click on the test option and give an event name and give an empty json in the Event Json, since we are just creating an Instance we do not need a json for testing. Save the event and click on Test option.
We can test the code by creating our own custom test event.
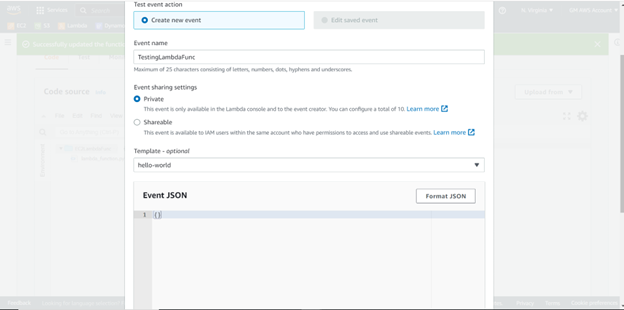
You can check the function logs to get the instance id of the newly created EC2 instance.
You can verify the details of the created instance in the Instances service in the AWS console using this instance id.
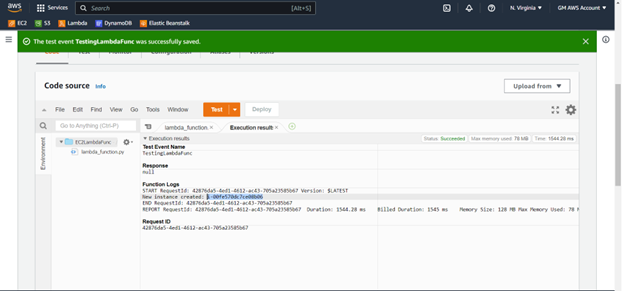
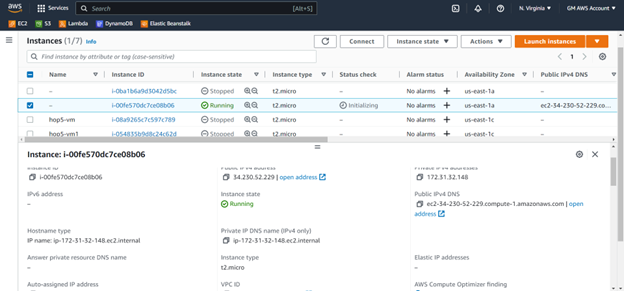
We have seen in detail how to create an EC2 instance using AWS Lambda functions. By making few changes to the above code we can also create multiple instance with the same configuration within minutes and without any bugs.