Welcome to Docker Series – Part 1
What is Docker, and Why do we need it?
Docker is a containerization tool that enables you to create, deploy, and run applications in isolated environments. This means that you can package an application with all its dependencies and ship it off to another machine without worrying about whether or not it will run properly.
Previously, we would have to deploy our applications on individual servers, requiring us to install all of the dependencies for each application on each server. This was time-consuming and often resulted in dependency conflicts. With Docker, we can create a container for each application and its dependencies. This way, we can deploy our applications quickly and easily, and we don’t have to worry about dependency conflicts.
Docker is popular because it is easy to use and solves the “it works on my machine” problem that often plagues developers. When you containerize an application, you can be sure it will run the same on any machine with Docker installed. Docker makes it easy to microservice applications. A microservice is an application that is composed of small, independent services. Each service is responsible for a single task, and they all work together to form a complete application. Docker makes deploying and managing microservices easy because each service can be run in an isolated environment. This makes it easy to update and scale individual services without affecting the other services in the application.
Docker is used to run containers on Kubernetes. Kubernetes is a container orchestration platform that allows you to manage and deploy containers. Kubernetes allows you to manage and deploy containers across a cluster of servers.
Getting started with Docker is easy. All you need is a computer with a Linux or Windows operating system and an internet connection. Once you have Docker installed, you can pull images from Docker Hub, a repository.
What is a Docker Container?
A Docker container is a standard unit of software that packages up code and all its dependencies, so the application runs quickly and reliably from one computing environment to another.
Docker containers are used to run software applications in isolated environments. This is different from virtual machines (VMs), which emulate a complete computer system. Instead, containers provide a way to run multiple isolated systems on a single host.
Docker containers are often compared to shipping containers. Just as a shipping container can be used to ship goods around the world, a Docker container can be used to ship software applications.
Docker containers are self-contained and include all the necessary files and dependencies for an application to run. This makes them portable and easy to deploy. Docker containers are isolated from each other and the host operating system. This enables them to run independently and securely.
What is a Docker Image?
A Docker image is a read-only template that contains a set of instructions for creating a container. It is a lightweight, standalone, executable package of software that includes everything needed to run an application: code, runtime, system tools, system libraries, and settings. Container images become containers at runtime, and in the case of Docker containers – images become containers when they run on Docker Engine.
Installing Docker on Ubuntu
If you want to experiment with Docker, install it on your local machine. Alternatively, You can also provision a VM on Azure or AWS.
Provision Your Virtual Machine (Azure)
Provision Your Virtual Machine (AWS)
To install Docker on your virtual machine:
Connect to your virtual machine using Bitvise and open a terminal window, then update the list of available packages to the latest version
sudo apt-get update -y
Once all the packages are up to date, install the docker package using the below command:
sudo apt-get install docker.io -y
Once the package is installed, you can check the status of the docker service:
sudo service docker status
You can also start/stop the service using the below commands:
sudo service docker start
sudo service docker stop
Add the user to the docker group so you can execute Docker commands without using sudo:
sudo usermod -aG docker $USER
Log out and log back in to pick up the new group permissions.
Verify that the installation was successful by running the following command
The below command runs the “hello-world” image in a Docker container.
docker run hello-world
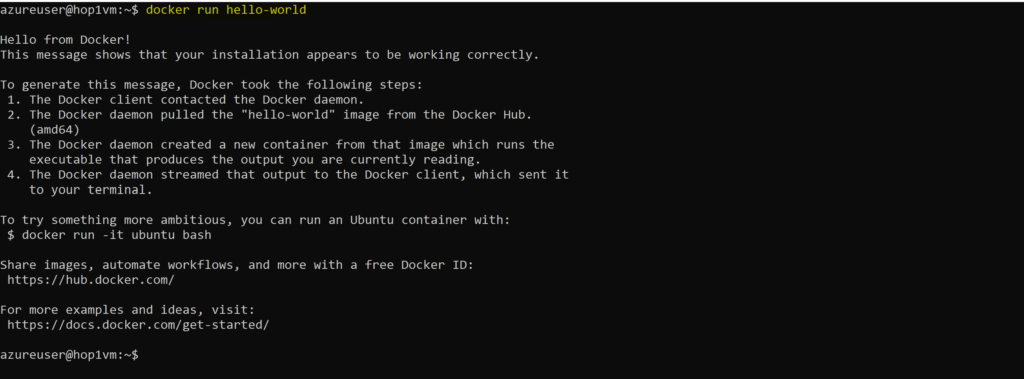
Running a Python Docker Image
Now, let’s run Python using docker.
You can pull the Python Image from the docker hub to your computer with the following command
docker pull python
Once you have pulled the Python image to your computer, you can run it with the following command:
docker run -it python
This will start a container based on the python image. You will be placed into a shell inside of the container. From here, you can run any commands that you would normally run inside a python shell.
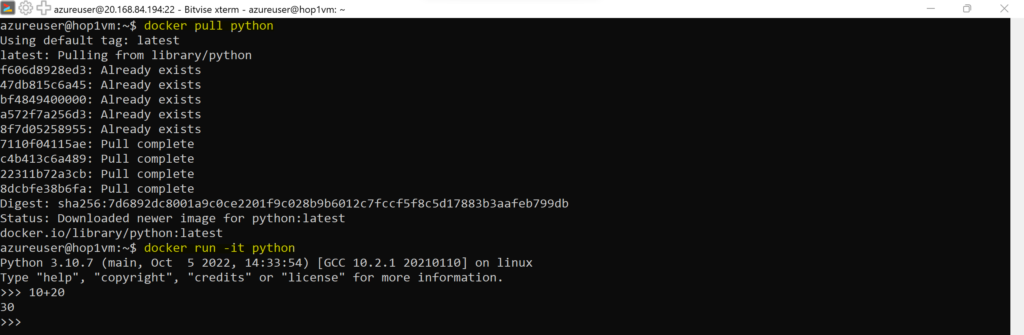
Some useful docker commands
Note: You can provide any docker image in the below commands in place of <image name>.
You can access the Docker Image Repository here.
To check the docker version:
docker version
To pull an image from the docker repository:
docker pull <image name>
To push an image that you have created to the Docker Hub:
docker push <image name>
To start a new container and attach it to the terminal in interactive mode (-it):
docker run -it <image name>
To list all the Docker images that are available on the system:
docker images

To list all of the containers that are currently running on the system.
docker ps
