In this article, we’re going to create a REST API gateway endpoint which is going to invoke a lambda function and return JSON back to the client by doing the following:
- Creating a API Gateway and Lambda function.
- Establishing a connection between Lambda function and API gateway.
- Managing access to the function with AWS Identity & Access Management (IAM) policies
Why AWS Lambda? The Lambda platform allows you to create and configure a function that runs in the AWS environment. An overwhelming benefit of these functions is that you only pay for the resources needed to execute the function, making them an attractive option for organizations looking for a scalable and cost-effective way to add cloud functionality.
What is REST API? A REST API (also known as RESTful API) is an application programming interface (API or web API) that conforms to the constraints of REST architectural style and allows for interaction with RESTful web services. It is a set of definitions and protocols for building and integrating application software. It’s sometimes referred to as a contract between an information provider and an information user-establishing the content required from the consumer (the call) and the content required by the producer (the response). In other words, if you want to interact with a computer or system to retrieve information or perform a function, an API helps you communicate what you want to that system so it can understand and fulfill the request.
Overview
First, we will set up a REST API called transactions having a configuration such that it forwards requests to the Lambda function. Then we will create a Lambda function named Transaction Handler and use python to code up the Lambda function in such a way that it can be invoked by API gateway. We will invoke the endpoint(GET) from our browser through the API URL and we will check the response in cloud watch to see if our deployment was successful. The following Figure 1 illustrates how the components of our deployment fit together:
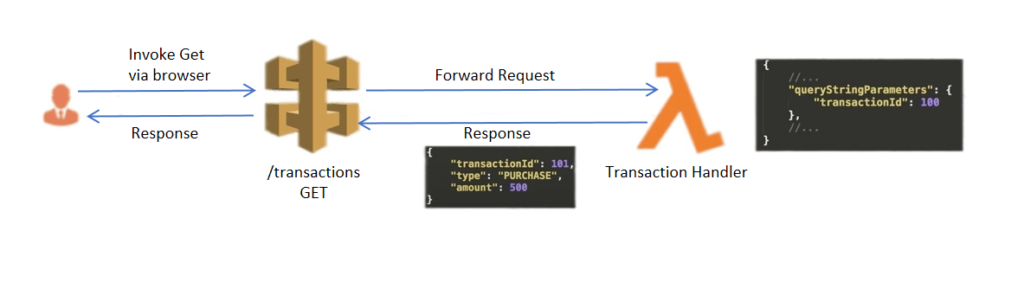
Step 1 – Build a REST API
- In the AWS management console, navigate to API gateway and select REST API and click on Build.
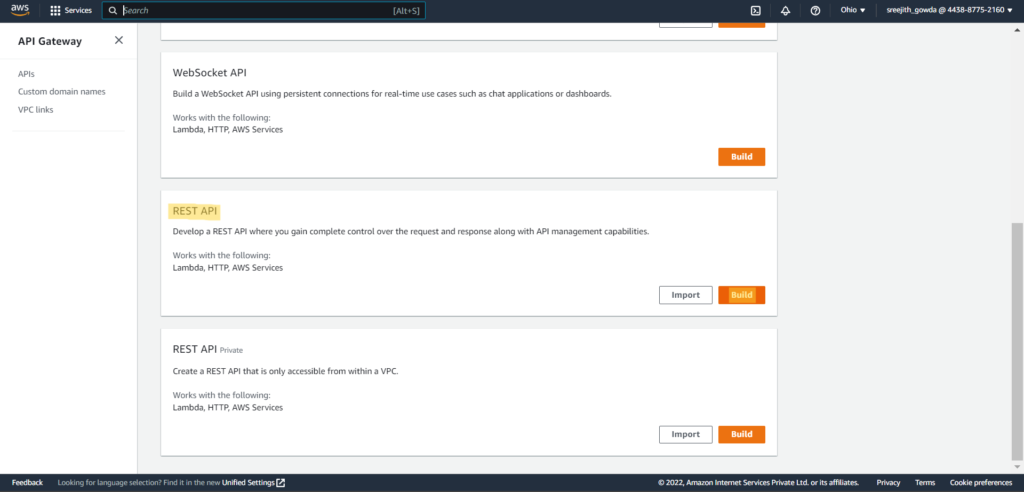
- In the API Gateway page, under protocol select REST.
- Also select NEW API in the Create new API section.
- In the Settings, give a name to your API and in this case it is “TranscationApis”.
- For Endpoint Type, Select Regional and click on Create API.
Why Regional API? The regional API endpoint is intended for clients in the same region and it is is intended to serve a small number of clients with high demands. Most importantly, reduces connection overhead.
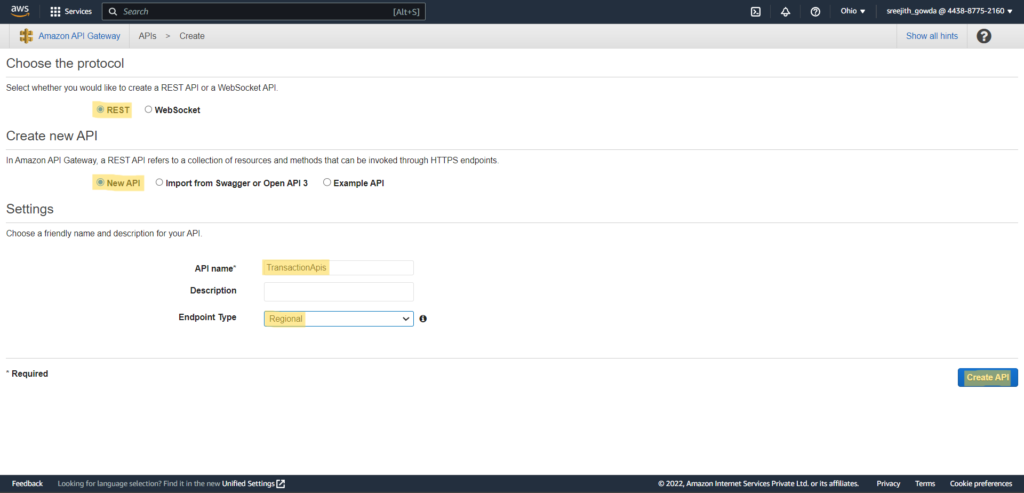
- Once the API is created we need to create resource in order to create a method which invokes lambda function.
- In the API gateway page, Under Actions, click on Create Resource.
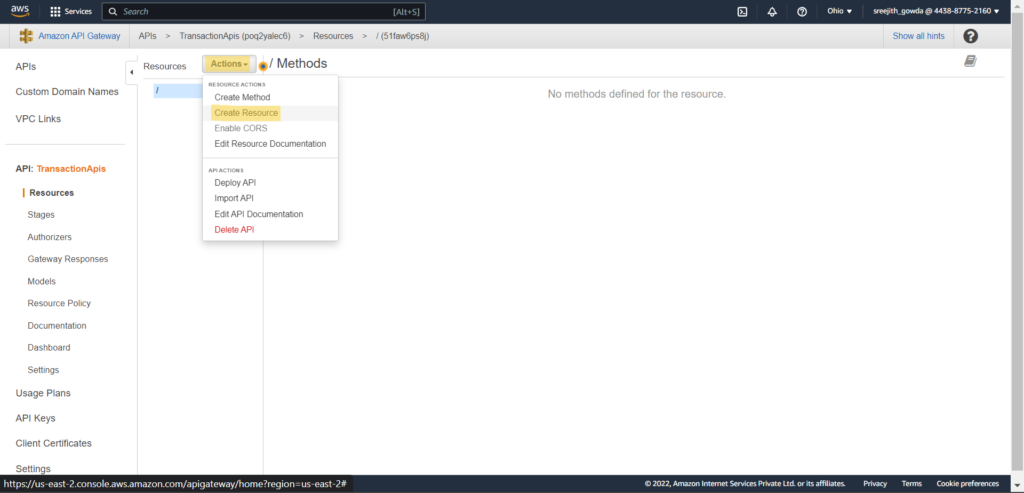
- In the next section, give a resource name and path. In this case it is “transactions” and then select Create Resource.
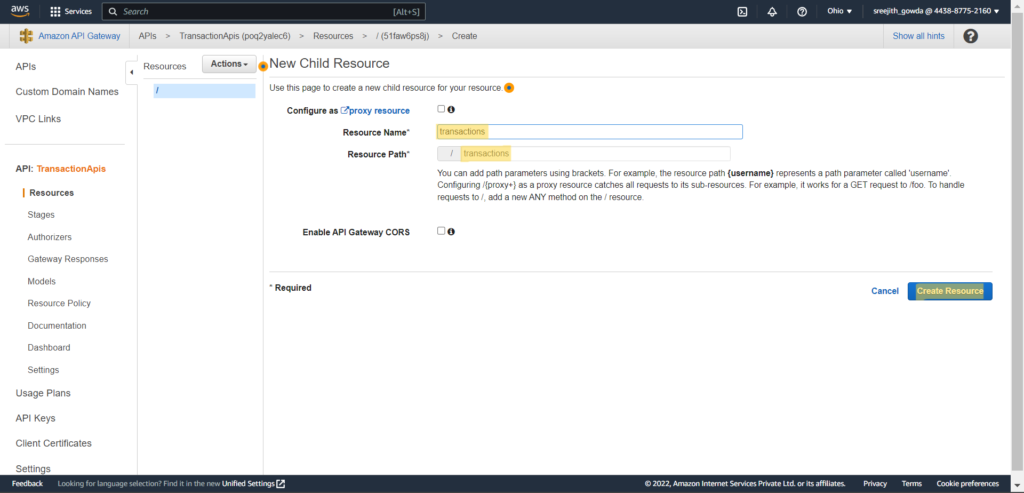
Step 2 – Create a Lambda function.
- In the AWS management console, navigate to Lambda and select Create Function as shown in the Figure .
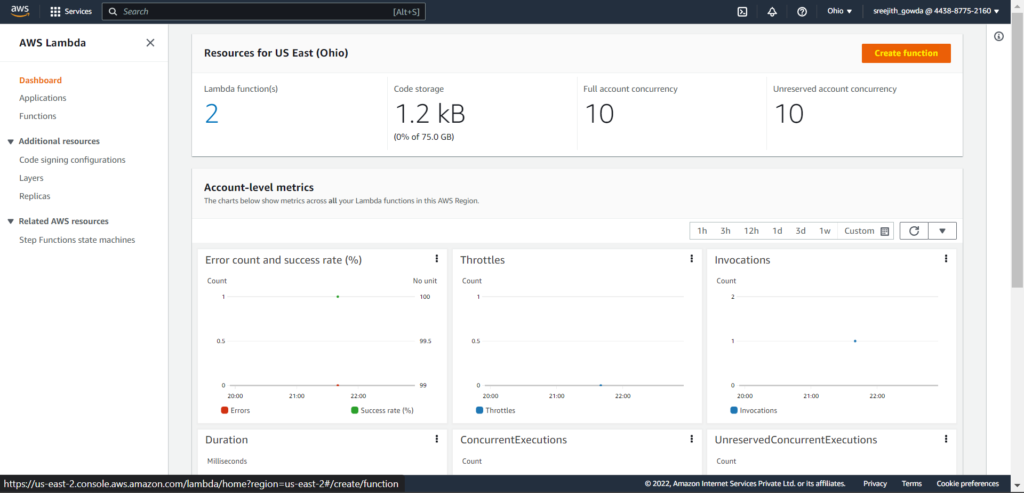
- Since we are creating the function on our own select “Author from scratch”.
- Enter a Function name. In this case it is “TransactionProcessor”.
- Specify the runtime environment (i.e., the version of python to use) and leave everything else on default and select Create Function.
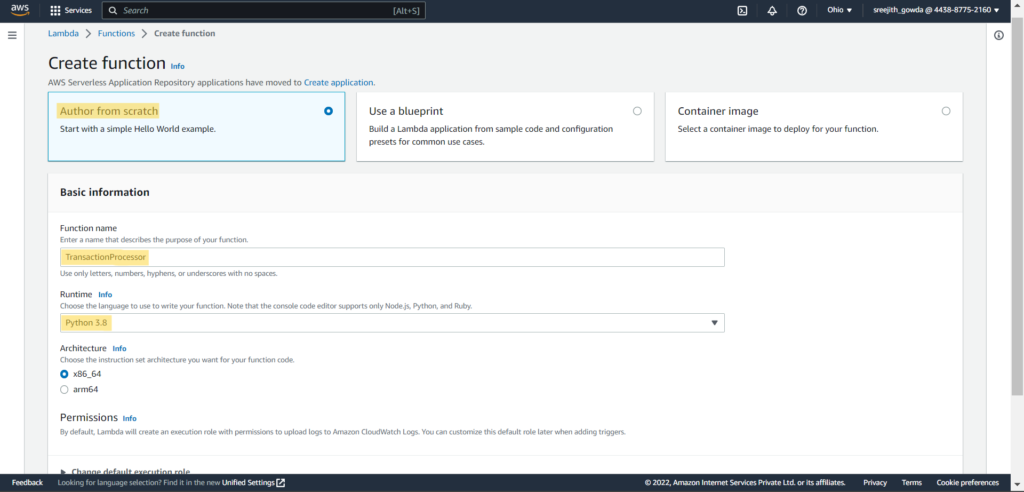
- Navigate to the Code tab below the function overview.
- Select Lambda_Function.py and view the code for the Lambda.
- Copy the code below, and replace the Lambda code with it:
import json
print('Loading function')
def lambda_handler(event, context):
#1. Parse out query string parameters
transactionId = event['queryStringParameters']['transactionId']
transactionType = event['queryStringParameters']['type']
transactionAmount = event['queryStringParameters']['amount']
print('transactionId=' + transactionId)
print('transactionType=' + transactionType)
print('transactionAmount=' + transactionAmount)
#2. Construct the body of the response object
transactionResponse = {}
transactionResponse['transactionId'] = transactionId
transactionResponse['type'] = transactionType
transactionResponse['amount'] = transactionAmount
transactionResponse['message'] = 'Hello world, This is lambda land'
#3. Construct http response object
responseObject = {}
responseObject['statusCode'] = 200
responseObject['headers'] = {}
responseObject['headers']['Content-Type'] = 'application/json'
responseObject['body'] = json.dumps(transactionResponse)
#4. Return the response object
return responseObject
- The code is a function that parses out the query string parameters and constructs an object with relevant information.
- It constructs a response object that contains information about the transaction.
- The response object is constructed in four steps: 1) Parse out query string parameters 2) Construct the body of the response object 3) Construct http response object 4) Return the response object.
- At the end it returns the response object which contains the status code, headers, and body of the response.
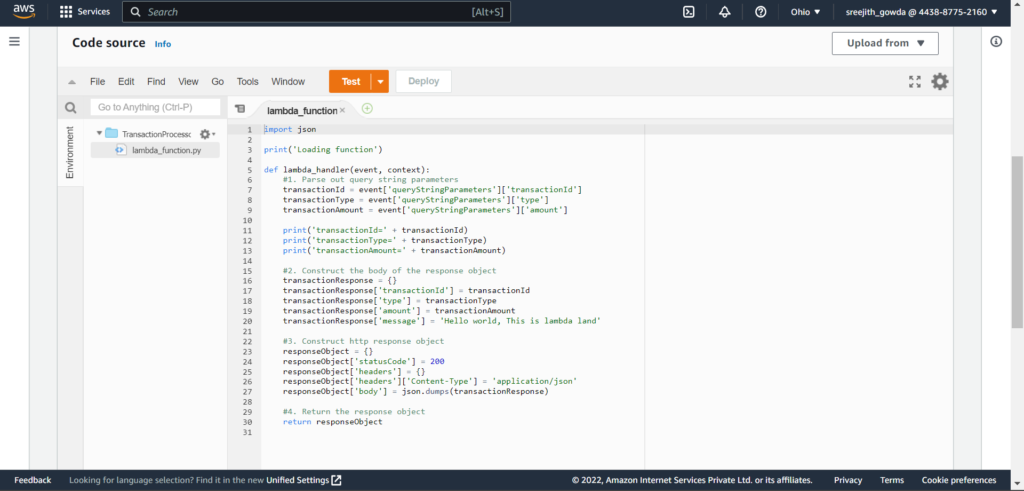
- Click on deploy to run the Lambda function.
Step 3 – Establish a connection between Lambda function and API gateway
- Go to the resources of the API which was created earlier, under actions click on Create method.
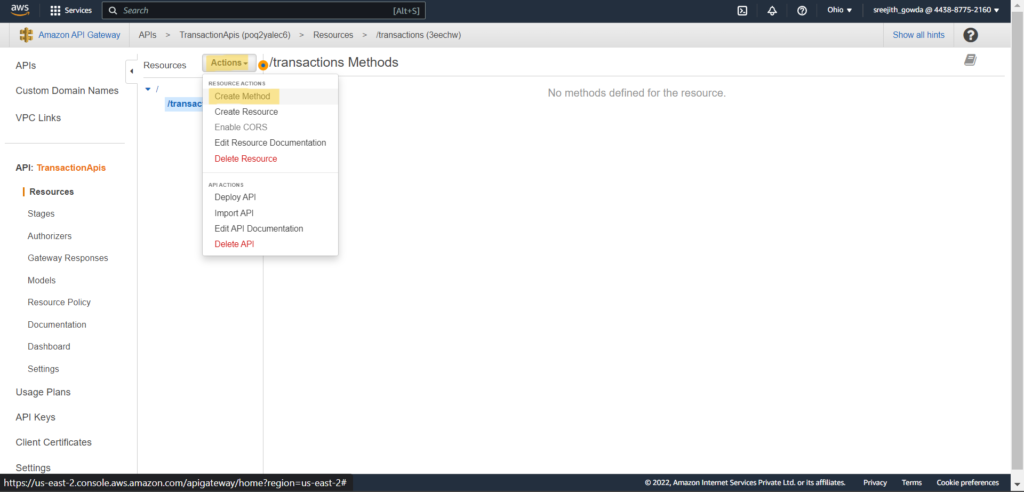
- Now select “GET” from the dropdown menu.
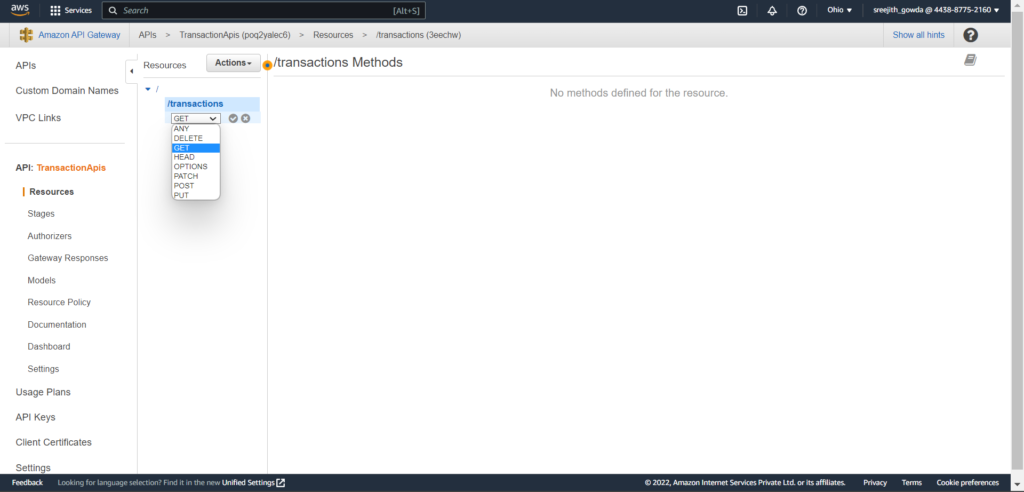
- Set the Integration type to Lambda Function.
- Check the box for Use Lambda Proxy integration.
Why Lambda proxy integration? Amazon API Gateway Lambda proxy integration is a simple, powerful, and nimble mechanism to build an API with a setup of a single API method. The Lambda proxy integration allows the client to call a single Lambda function in the backend. The function accesses many resources or features of other AWS services, including calling other Lambda functions. In Lambda proxy integration, when a client submits an API request, API Gateway passes to the integrated Lambda function the raw request as-is, except that the order of the request parameters is not preserved. This request data includes the request headers, query string parameters, URL path variables, payload, and API configuration data. The configuration data can include current deployment stage name, stage variables, user identity, or authorization context (if any). The backend Lambda function parses the incoming request data to determine the response that it returns.
- The region is the same one where you defined your functions and in this case it is US-east-2.
- Select your Lambda function which you created earlier and click save.
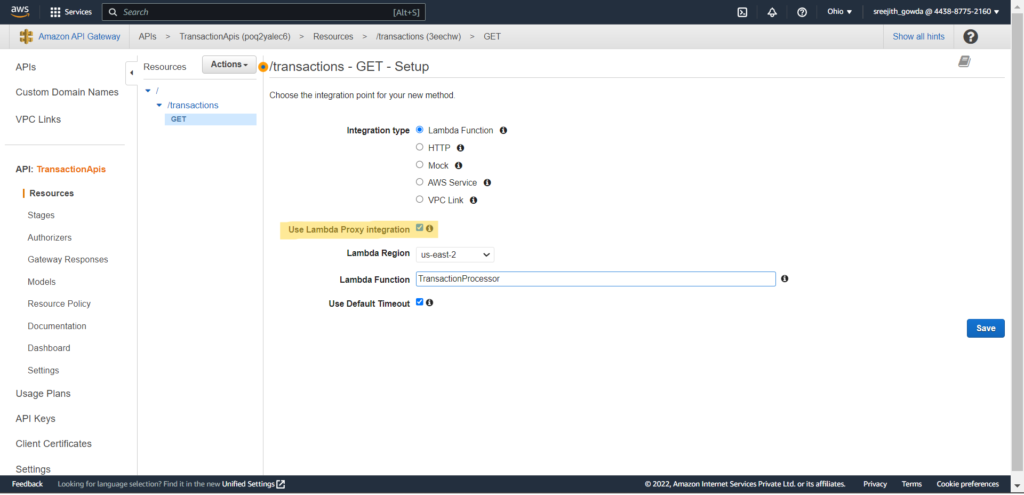
- Now that we have established a connection between our Lambda function and API gateway, we can head over to Lambda function page to verify the connection.
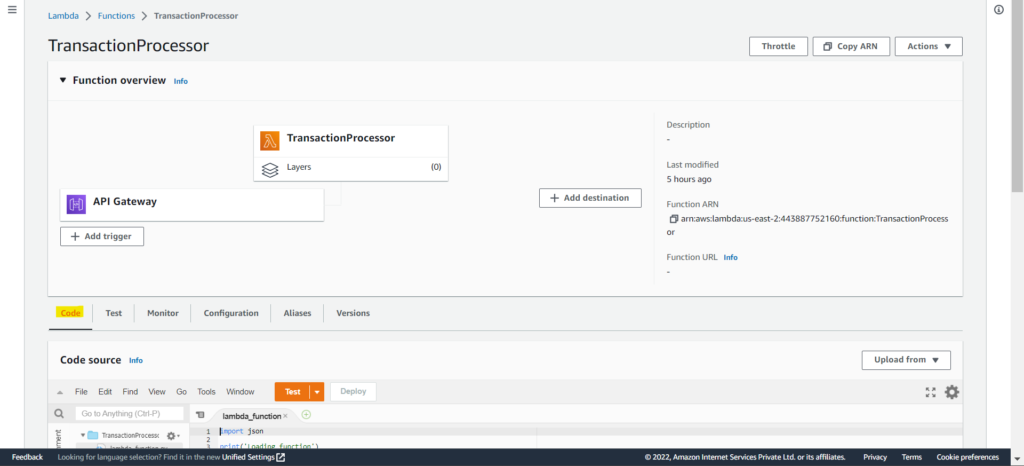
- After the verification of the above process return back to API gateway and deploy the API.
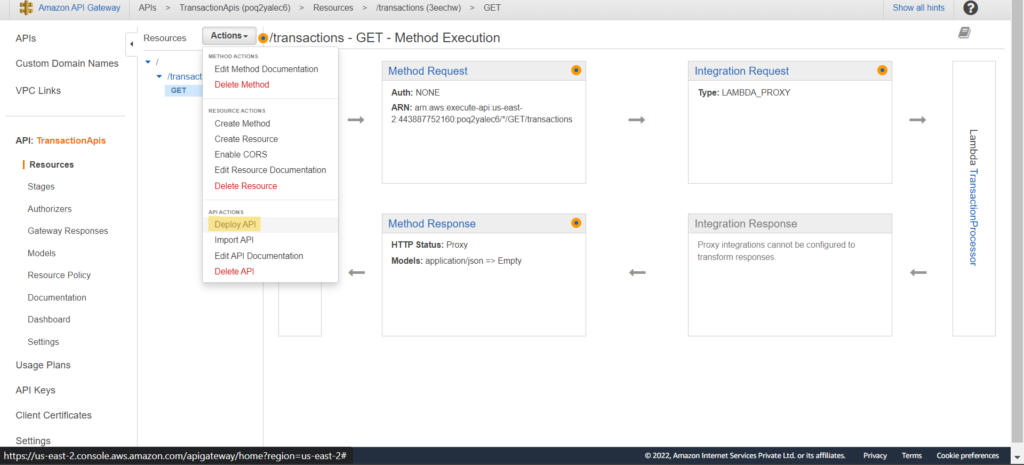
- Specify the stage of your API and here in this case it is test and click on deploy.
- Upon successful completion of the above steps, we will get the Invoke URL.
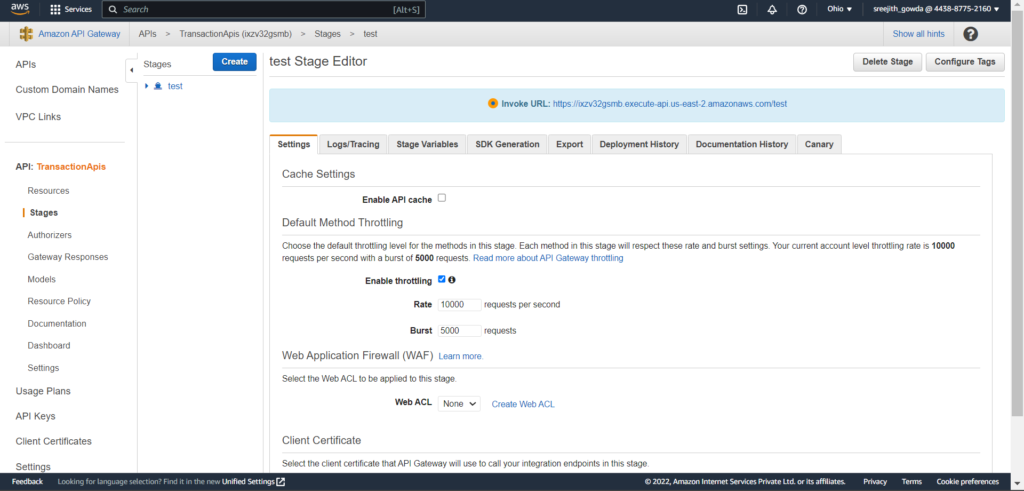
- Copy the URL to your browser and specify the ID, type and amount along with the URL.
- In this case, the transactionId is 5, transactionType is PURCHASE and transactionAmount is 500.
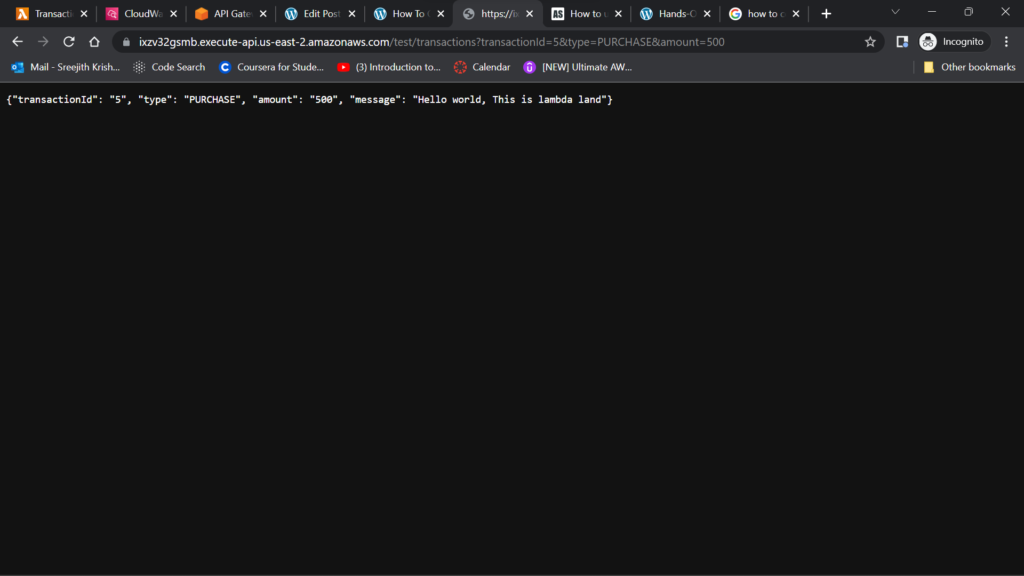
- In order to verify the response, head over to Cloud watch and check the time stamp along with the message received. Here in this case, the transactionId is 5, transactionType is PURCHASE and transactionAmount=500.
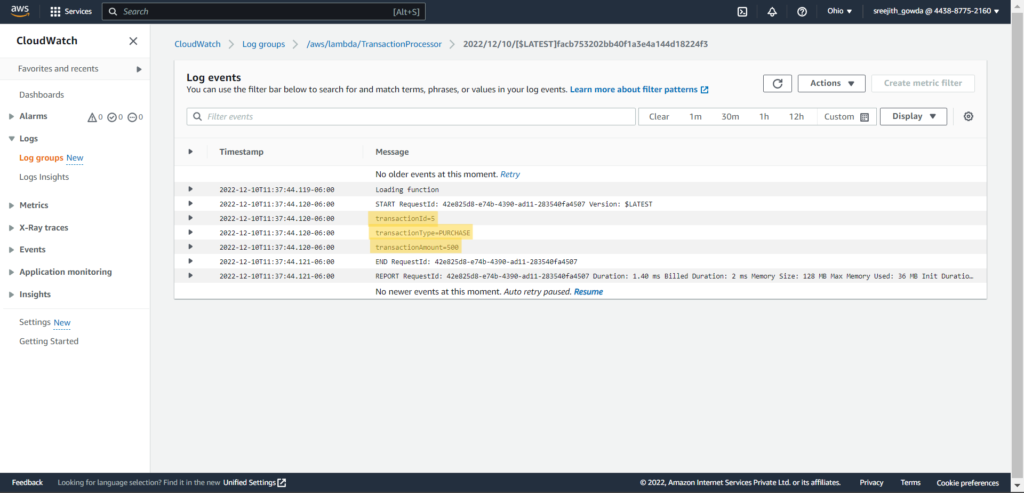
Python And AWS Lambda – Wrapping Up
Remember that AWS charges you for everything that’s running on your account, so when you’re done with the example in this article, don’t forget to clean up the API, Lambdas, and other infrastructure. The simplest way is:
- On the API Gateway homepage, select the API Gateway you created, and choose Delete from the Actions dropdown.
- From the CloudFormation webpage, select the stack you created and click on the Delete option at the top of the page.
- AWS should remove everything within a few minutes.