Objective: To understand the working of Timer Trigger. In addition to building and invoking a python function schedule to print every 15s.
Prerequisite:
- Python 3.8 – Python 3.10 (Python 3.11 is not supported yet.)
- Azure Core Tools – Download azure core tools from here
- VS Code
Step 1: Open VS Code
Head to extensions and install Azure Tools extensions. After which you would be prompted to sign into your Azure Account.
Then from your local PC or cloud PC, start VS Code. Open a new project folder or within your existing project folder > Choose Python as your language > Timer Trigger (enter the trigger name) > Trigger Function Name (should be globally unique)
After entering the function name you are required to mention the time in CRON expression, for 15s enter “*/15 * * * * *”
NCRONTAB expressions
Azure Functions uses the NCronTab library to interpret NCRONTAB expressions. An NCRONTAB expression is similar to a CRON expression except that it includes an additional sixth field at the beginning to use for time precision in seconds:
{second} {minute} {hour} {day} {month} {day-of-week}
Each field can have one of the following types of values:
Type | Example | When triggered |
A specific value | 0 5 * * * * | Once every hour of the day at minute 5 of each hour |
All values (*) | 0 * 5 * * * | At every minute in the hour, beginning at hour 5 |
A range (- operator) | 5-7 * * * * * | Three times a minute – at seconds 5 through 7 during every minute of every hour of each day |
A set of values (, operator) | 5,8,10 * * * * * | Three times a minute – at seconds 5, 8, and 10 during every minute of every hour of each day |
An interval value (/ operator) | 0 */5 * * * * | 12 times an hour – at second 0 of every 5th minute of every hour of each day |
To specify months or days you can use numeric values, names, or abbreviations of names:
- For days, the numeric values are 0 to 6 where 0 starts with Sunday.
- Names are in English. For example: Monday, January.
- Names are case-insensitive.
- Names can be abbreviated. Three letters is the recommended abbreviation length. For example: Mon, Jan.
I used this link to help me schedule timing for my function in terms of NCRONTAB expressions.
Review your steps and tap Enter, which shall results in opening a new workspace.
Step 2: Explore the workspace
You can head to function.json file and locate the name, type and schedule period in the NCRON expression.
{
"scriptFile": "__init__.py",
"bindings": [
{
"name": "mytimer",
"type": "timerTrigger",
"direction": "in",
"schedule": "*/15 * * * * *"
}
]
}
__init__.py
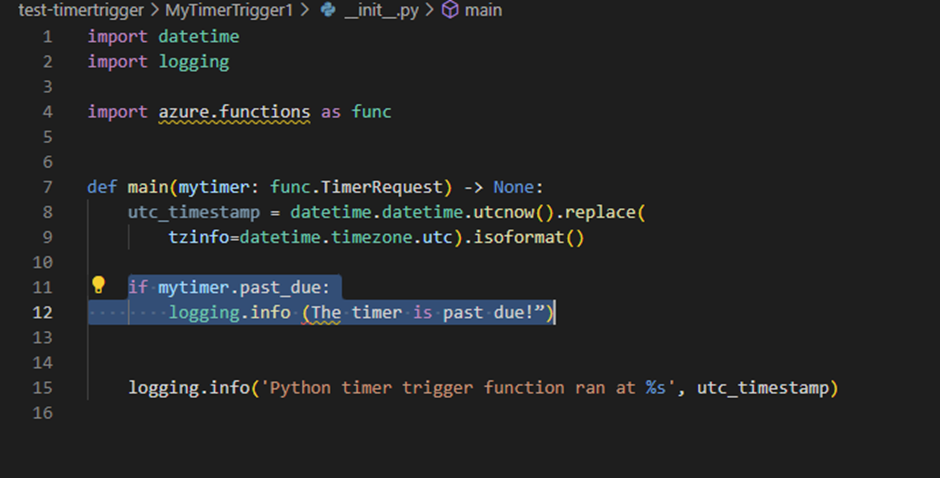
We then move to the main function “__init__.py”, over here we will replace the above highlighted code with a simple print text that helps us to determine the working of trigger schedule. The role of this trigger function is to print the result every 15s that our function gets triggered. Our replacement code allows us to trigger the function every 15 seconds and print it in inside the terminal.
It should look like the below attached code,
import datetime
import logging
import azure.functions as func
def main(mytimer: func.TimerRequest) -> None:
utc_timestamp = datetime.datetime.utcnow().replace(
tzinfo=datetime.timezone.utc).isoformat()
print("\n My Function executing in 15 seconds using Timer Trigger")
logging.info('Python timer trigger function ran at %s', utc_timestamp)
After this step, please ensure you have Azure Core Tools installed and run the program by clicking F5.
Step 3: Azure Core Tools
Upon running the program, Azure requests access to storage account, you can use the existing one or create a new one.
Next, Select the region, it is highly recommended to choose your location or the one nearest to you.
Once completed, the function should open the terminal and execute our print statement every 15s. You can review the below image as your expected output:-
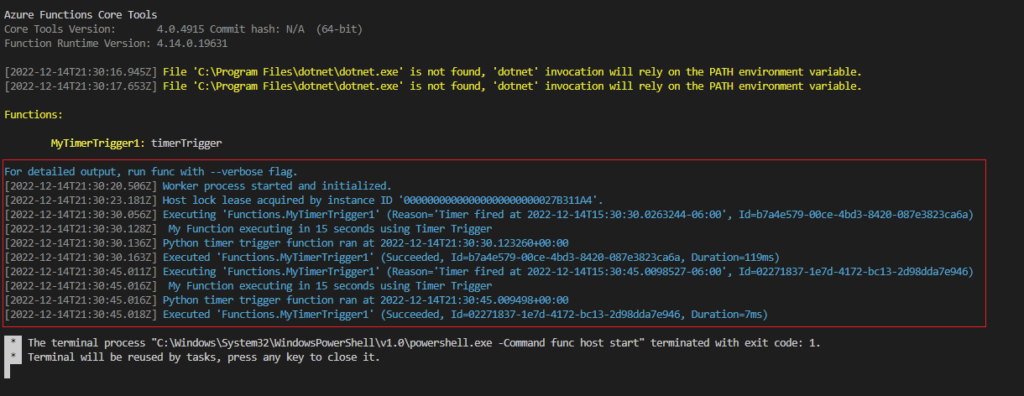
Eventually this can be guiding stream for some below uses cases:
Postponing Email Popup
Adjusting the bounce rate – this is useful for digital marketers. (Example: creating the pop ups bots on website)
Combining with another trigger
And that’s how you set up your timer trigger. I hope the steps were clear and this was easy walkthrough for understanding the working of timer trigger. Please comment below letting me know your thoughts.